The Challenge: Why Businesses Need Serverless Computing
As businesses grow in the digital era, the demand for real-time processing, automation, and high-performance applications continues to increase. Whether an e-commerce platform handles orders, a fintech company processes transactions, or an analytics firm generates reports, businesses need reliable, scalable, and cost-effective solutions to execute backend operations.
The Growing Challenge of Managing Infrastructure
Traditionally, companies relied on dedicated servers or virtual machines (VMs) to handle business operations. However, as workloads increased, they faced significant challenges:
- High infrastructure costs: Paying for idle resources even when applications werenβt running.
- Complex maintenance: Server management, patching, and scaling became a full-time job.
- Slow scalability: Handling traffic spikes required manual intervention or over-provisioning.
- Latency & inefficiencies: Backend jobs like data processing, automation, and API requests slowed customer experience.
To address these issues, businesses began searching for a solution that eliminates infrastructure overhead, scales automatically, and reduces costs. One solution is AWS Lambda, a serverless computing service that handles backend processing independently of server management.
The Serverless Revolution: How AWS Lambda Transforms Business Operations
AWS Lambda allows businesses to execute code responding to events without provisioning or managing servers. Instead of maintaining dedicated infrastructure, Lambda executes functions only when needed, scaling automatically and charging only for execution time.
π Why Businesses Are Switching to AWS Lambda:
β
No servers to manage: No provisioning, patching, or infrastructure concerns.
β
Automatic scaling: Handles millions of requests per second effortlessly.
β
Event-driven execution: Triggers on demand, saving costs on idle resources.
β
Seamless AWS integration: Works with API Gateway, DynamoDB, S3, RDS, and more.
β
Cost savings: Businesses only pay for execution time, reducing wasted resources.
Example: Imagine a business that needs to process thousands of customer orders daily. With traditional servers, they would need to maintain and scale infrastructure continuously. However, AWS Lambda’s order processing function executes when triggered, eliminating wasted resources and cutting costs significantly.
π Step-by-Step Guide: Deploying a Serverless Application with AWS Lambda
1. Creating an AWS Lambda Function with Python
AWS Lambda runs code in response to triggers, such as API requests, file uploads, or scheduled events.
Steps to Create a Lambda Function:
- Go to AWS Lambda Console β click Create Function.
- Choose “Author from scratch” and name your function.
- Select Python 3.x as the runtime environment.
- Define an IAM role with permissions to access other AWS services.
- Write and deploy your Lambda function.
Example: Python Function
import json
def lambda_handler(event, context):
return {
'statusCode': 200,
'body': json.dumps("Hello from AWS Lambda!")
}
Result: Lambda is now ready to execute code automatically without needing a dedicated server.
2. Exposing AWS Lambda via API Gateway
Businesses often need to expose Lambda functions as API endpoints for web and mobile applications. AWS API Gateway helps route external requests securely.
Steps to Connect API Gateway with Lambda:
- Go to API Gateway Console β click Create API.
- Select REST API β Choose New API.
- Click Create Resource β Define a lambda-endpoint path.
- Choose ANY HTTP method β Set the integration type as Lambda Function.
- Deploy the API β Generate the invoke URL.
Result: Lambda is now accessible via an API endpoint, making it callable from web or mobile applications.
3. Storing Data in DynamoDB for Serverless Data Persistence
Many serverless applications need persistent data storage. AWS DynamoDB provides a fully managed, scalable NoSQL database that integrates seamlessly with Lambda.
Steps to Connect Lambda with DynamoDB:
- Go to AWS DynamoDB Console β Click Create Table.
- Set a Table Name (e.g., Orders) and Primary Key (OrderID).
- Add IAM permissions to the Lambda function to access DynamoDB.
- Modify the Lambda function to store data in DynamoDB.
π Example: Python Code in Lambda to Write Data to DynamoDB:
import boto3
import json
dynamodb = boto3.resource('dynamodb')
table = dynamodb.Table('Orders')
def lambda_handler(event, context):
order = {
'OrderID': event['order_id'],
'Customer': event['customer_name'],
'TotalAmount': event['amount']
}
table.put_item(Item=order)
return {
'statusCode': 200,
'body': json.dumps("Order saved successfully!")
}
Result: The serverless function now stores data in DynamoDB, eliminating the need for a dedicated database server.
This architecture demonstrates a serverless workflow using AWS API Gateway, AWS Lambda, and Amazon DynamoDB to process user requests without managing infrastructure, as shown in Figure 1.
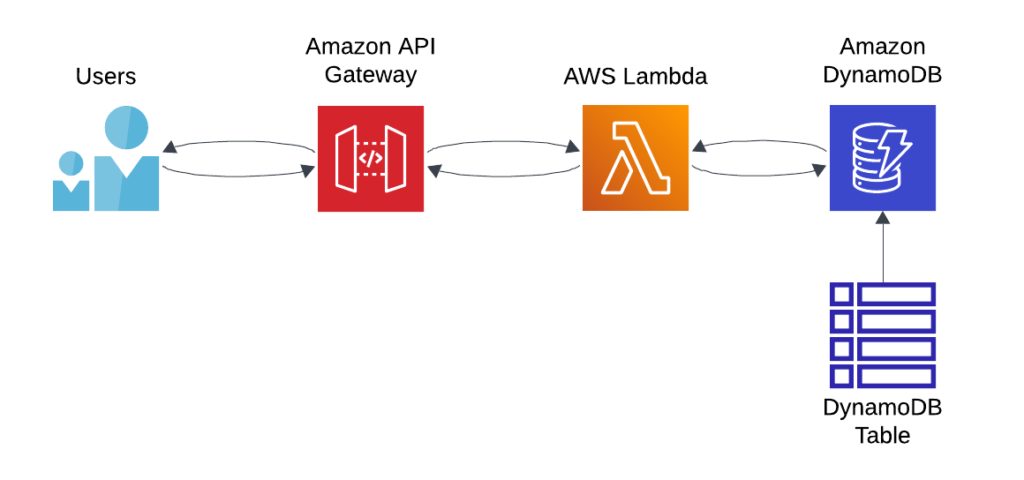
Figure 1: Serverless Architecture
Business Benefits of Serverless Architecture
By adopting AWS Lambda, API Gateway, and DynamoDB, businesses achieve:
- Cost Efficiency: No infrastructure costs, only pay for execution time.
- High Scalability: Automatically scales with traffic demand.
- Simplified Maintenance: No need for server patching, updates, or capacity planning.
- Faster Development: Focus on business logic instead of managing infrastructure.
Example Use Cases:
- E-Commerce: Processing orders, handling payments, tracking inventory.
- IoT Applications: Real-time data processing from connected devices.
- Chatbots & AI Services: Event-driven automation without infrastructure overhead.
- Automated Reports & Notifications: Background tasks such as sending emails and processing analytics.